Apache+Python 實作線上聊天室
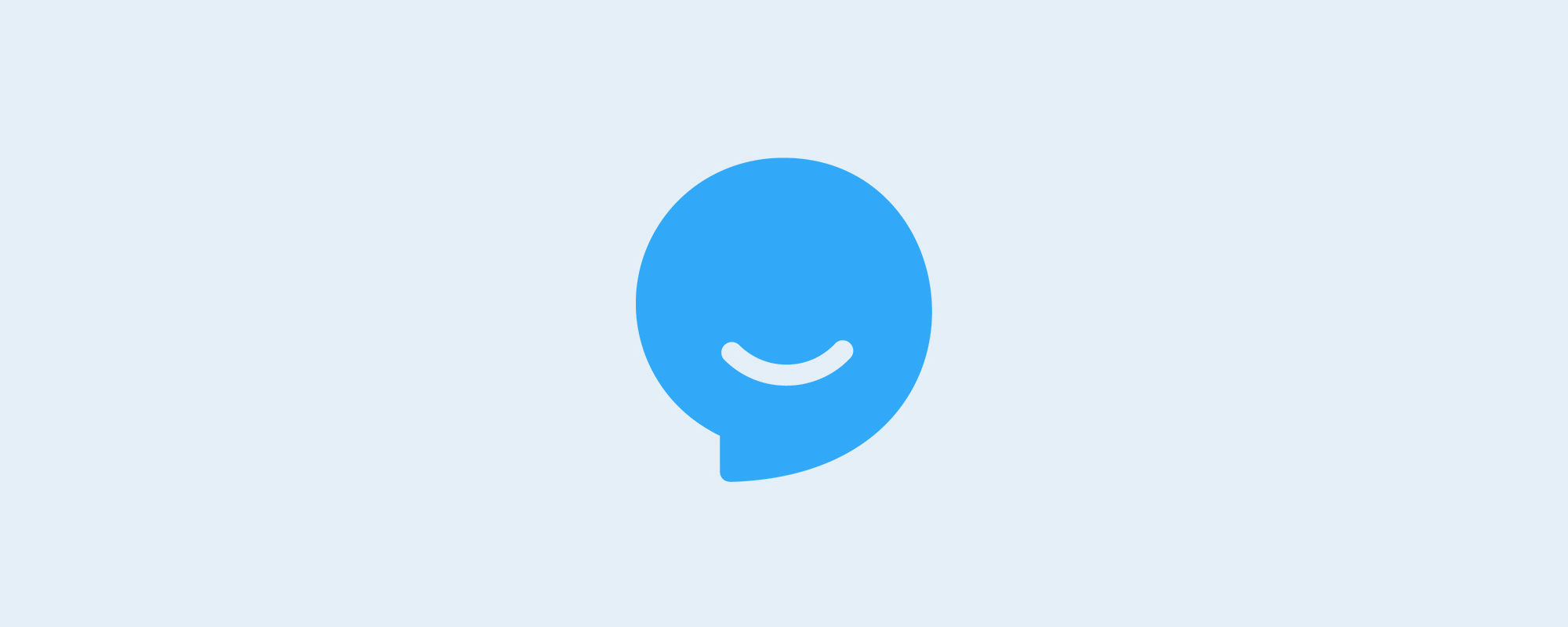
前言
班導因公務出差,所以請我幫忙代課,對象是日間部大一、夜間部大二。
我使用 XAMPP 連接後端 Python 程式架設聊天室,以此作為上課教材。
實作過程
前端 HTML
指定文字編碼為 UTF-8,並導入 jQuery,建立一個textarea
、兩個input
與一個button
。
<head>
<meta charset="utf-8">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
</head>
<body>
<div>
<textarea id="output_txt" style="width:650px; height:650px;"></textarea>
</div>
<div>
<input type="text" id="name" placeholder="暱稱" style="width:100px;">
<input type="text" id="msg" placeholder="說點什麼?" style="width:500px;">
<button id="btn">送出</button>
</div>
</body>
前端 JS
收到後端的回覆後,首先清空msg
的內容後,將回覆更新於output_txt
,並將其滾動至底部。
在input
元件上監控keydown
事件,若按下Enter
(JS Code:13),就模擬btn
被按下。
最後每秒呼叫後端reflash.py
,藉以隨時更新output_txt
。
<script>
$("#btn").on("click", function(){
$.ajax({
url: "./chat.py",
type: "post",
datatype: "json",
data:
{
"name": $("#name").val(),
"msg": $("#msg").val()
},
success: function(response){
$("#msg").val("");
$("#output_txt").val(response.output_txt);
$("#output_txt").scrollTop($("#output_txt")[0].scrollHeight);
}
});
});
$("input").keydown(function (event){
if (event.which == 13)
{
$("#btn").click();
}
});
setInterval(function(){
$.ajax({
url: "./reflash.py",
success: function(response){ $("#output_txt").val(response.output_txt); }
});
}, 1000);
</script>
後端 Python
chat.py
#!C:/Python37/python
import json, cgi
fs = cgi.FieldStorage()
name = fs.getvalue("name")
msg = fs.getvalue("msg")
with open("./msgs.txt", "a") as file:
file.write("{" + name + "}:" + msg + "\n")
with open("./msgs.txt", "r") as file:
response = {}
response["output_txt"] = file.read()
print("Content-Type: application/json\n\n")
print(json.dumps(response))
reflash.py
#!C:/Python37/python
import json
with open("./msgs.txt", "r") as file:
response = {}
response["output_txt"] = file.read()
print("Content-Type: application/json\n\n")
print(json.dumps(response))
貼文底端